Have you ever wanted to copy a file to multiple directories? Well, you can do so with just one simple command. You can send a file to multiple directories at the same time pretty easily. In this case, we do it with the tee command.
This is also a 'short' article. I haven't done one of those in a while and this seemed like a fine time to do one. So, I did one...
linux-tips.us
Also, this time around I give some explicit instructions. If you follow those instructions, all things should become clear. That was the best way I could think of to explain this process. So, if you're new to this command (the tee command) you can learn by doing rather than learning by being told.
I think it worked well enough.
This is also a 'short' article. I haven't done one of those in a while and this seemed like a fine time to do one. So, I did one...
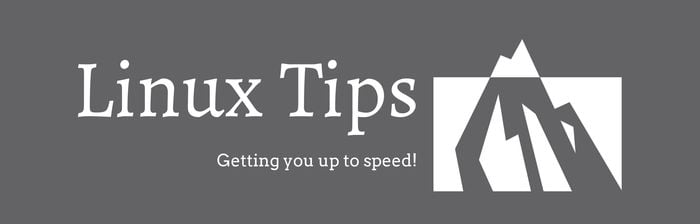
Short: Move A File To Multiple Directories • Linux Tips
Today's article should be a fairly short article, where I take the chance to show you how to move a file to multiple directories.

Also, this time around I give some explicit instructions. If you follow those instructions, all things should become clear. That was the best way I could think of to explain this process. So, if you're new to this command (the tee command) you can learn by doing rather than learning by being told.
I think it worked well enough.